What is Variables in Python A Detailed Guide
BlogBefore Starting on any programming journey, understanding what a variable is in Python is fundamental. In this tutorial, I break everything down so you understand the types of variables and how to make them, and also show you rules of governing the use. After reading this article, you will already have a general understanding of how to work with variables in Python and why they are important in coding.
Step 1 Variables Defined
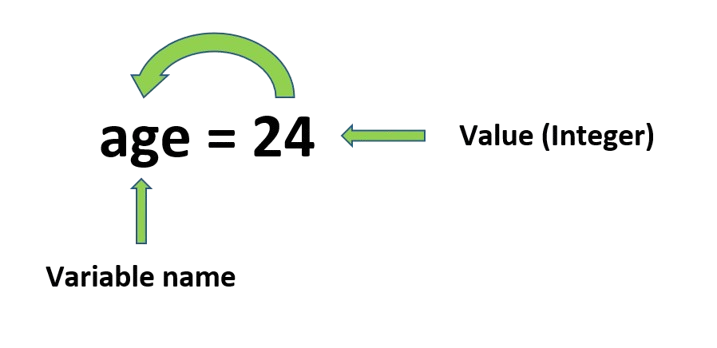
Variables in Python are used to represent named memory locations that store data. Think of them as containers holding information that you can refer to and manipulate throughout the program. The variable has versatility because it stores various types of information.
I can, for example, declare a variable name and give it a value. Now, this feature implies that I am able to reference this variable elsewhere in the code since it calls for the value mentioned earlier. This form of programming flexibility is essential since it allows the manipulation of the data.
Step 2 Creation of Variables
All that needs to be done to make a variable in Python is to create a name for the variable and then, using an equal sign =
, assign it a value. Here’s what it looks like:
name = "Alice"
print(name)
Here name is a variable and it contains the value of the string, ‘Alice‘.
Step 3 Types of Variables
Python, on the other hand, allows different types of variables, which can be specified depending on the preference of the programmer. The major types include:
- String: A data type that is used to store.
- Integer: To store whole numbers.
- Float: For storing decimal values.
- Boolean: holds the values true or false.
Step 3.1 String variables
In the case of string variables, the text is enclosed in quotation marks. For example:
greeting = "Hello, AiTechBite!"
print(greeting)
Here, greeting is a string variable, with the string Hello, AiTechBite! assigned to it.
Step 3.2 Integer Variables of
Integer variables are relatively simple. They will store whole numbers without any decimal points. For example:
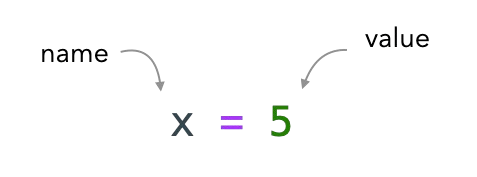
X = 30
print(X)
Here age is an integer variable and the value that it has is 30.
Step 3.3 Float Variables
Use a float variable if you want to store numbers for which decimal accuracy is needed. Here’s how to do that.
height = 5.9
print(height)
Here, height is a float variable holding the value 5.9.
Step 3.4 Boolean Data
Boolean variables are binary, meaning that they can only assume either of the two possible values: either True or False. For instance:
is_student = True
print(is_student)
This declares and initializes a Boolean variable called is_student that should let you know if the person is or isn’t a student the output of this now is TRUE.
Step 4 Data Entry by User
Another thing I can do with variables in Python is to create variables from user input. The command input() would enable me to ask questions of the user that acquire information from them. For example:
user_name = input("What is your name: ")
This code will elicit a response from the user by typing their name and place what is input into the variable userName.
Step 5 Print Out Variables
The value stored in a variable can be viewed through the print() function. Example:
print("Your name is", user_name)
It would echo out the string and value of user_name.
Step 6 Naming Conventions for Variables
When declaring variables, I have to follow the following rules:
- Variable names are allowed to start with either a letter or an underscore.
- They can’t start with a digit.
- No spaces allowed, use the underscores instead. Variable names are case sensitive.
- Do not use Python keywords for naming identifiers.
Step 7 Variables
Variables are assigned to in several ways within Python. I can assign a new value to an existing variable like this:
age = 30
age = 31 # Updating the value
The above code first assign 30 to age and then update its value to 31.
Step 8 Several Declares
Python allows me to assign values to more than one variable in a line of code. For example:
x, y, z = 1, 2, 3
That makes x 1 and y 2, as well as z 3 all together.
Step 9 Casting Data Type
Sometimes, I will wish to change the data type of a variable. The process whereby this is achieved is called typecasting. For example:
number = "5"
number = int(number) # Changing the string to int type
I’m parsing a string to an int here.
Step 10 Conclusion
You really need to grasp what a variable is within Python for programming purposes. I will, with the help of instructions within this guideline, be in a position to create, manipulate, and use variables in the process of developing my Python projects. The variable is considered as the backbone of any program because it has many dynamic data and logic-handling features. With every step further into learning programming, I master how to handle variables, thus being able to do more and more complex challenges with confidence.