A Step-by-Step Guide to Creating MongoDB Databases
BlogStep 1 Exploring MongoDB Databases ๐
As we delve into the world of MongoDB Databases one of the most crucial tasks is understanding how to create and MongoDB Databases. In this step-by-step tutorial, we’ll explore the various operations you can perform on databases and collections, ensuring you have a solid foundation to work with this powerful NoSQL database.
Step 2 Checking Existing MongoDB Databases ๐
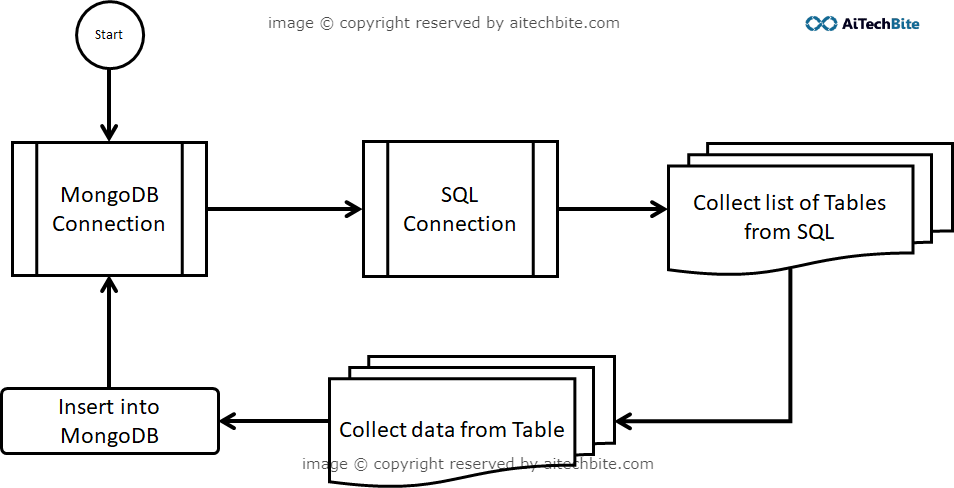
The first step is to check the databases that are already present in your MongoDB environment. You can do this by running the command show dbs
or show databases
. This will display a list of all the databases currently available in your MongoDB instance.
It’s important to note that if you haven’t created any databases yet, the list will only show the default databases, such as admin
, config
, and local
. These are system-level databases used by MongoDB for internal purposes.
Step 3 Creating a New MongoDB Databases ๐
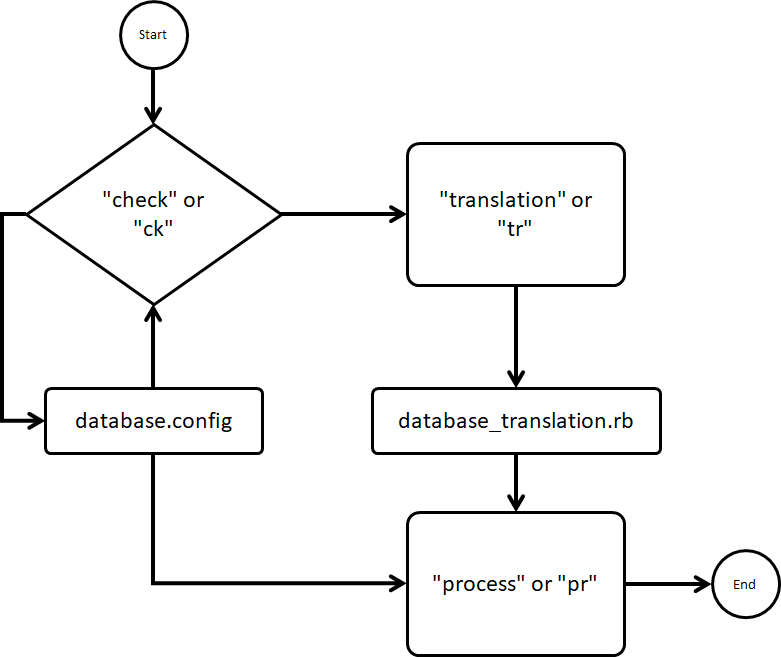
To create a new database, you can use the use
command followed by the name of the database you want to create. For example, if you want to create a database called “students”, you would run the following command:
use students
This command will create the “students” database if it doesn’t already exist. If the database already exists, the use
command will simply switch to that database.
Step 4 Deleting a MongoDB Databases ๐๏ธ
If you need to delete a database, you can use the db.dropDatabase()
command. This will remove the entire database, including all its collections and documents. Here’s an example:
db.dropDatabase()
Make sure you’re working in the correct database before running this command, as it will permanently delete all the data stored in that database.
Step 5 Creating Collections ๐
In MongoDB, data is stored in collections, which are similar to tables in a relational database. To create a new collection, you can use the db.createCollection()
command. For instance, to create a collection called “students” in the “students” database, you would run:
db.createCollection("students")
If you don’t want to explicitly create a collection, MongoDB will automatically create one when you insert the first document into it.
Step 6 Deleting Collections ๐๏ธ
To delete a collection, you can use the db.collection.drop()
command. This will remove the entire collection, including all the documents it contains. Here’s an example:
db.students.drop()
Make sure you’re working in the correct database and collection before running this command, as it will permanently delete all the data stored in that collection.
Step 7 Inserting Documents ๐
Now that we have a database and a collection set up, we can start inserting documents (data) into the collection. To insert a single document, you can use the db.collection.insertOne()
command. For example:
db.students.insertOne({
name: "Vinod",
age: 29,
course: "CS"
})
To insert multiple documents at once, you can use the db.collection.insertMany()
command and pass an array of document objects.
db.students.insertMany([
{name: "Vineet", age: 20},
{name: "Arjun", age: 30}
])
Step 8 Querying Documents ๐
Retrieving data from your MongoDB database is done using the db.collection.find()
command. This command allows you to filter and retrieve documents based on specific criteria. For example, to find all documents in the “students” collection, you can use:
db.students.find()
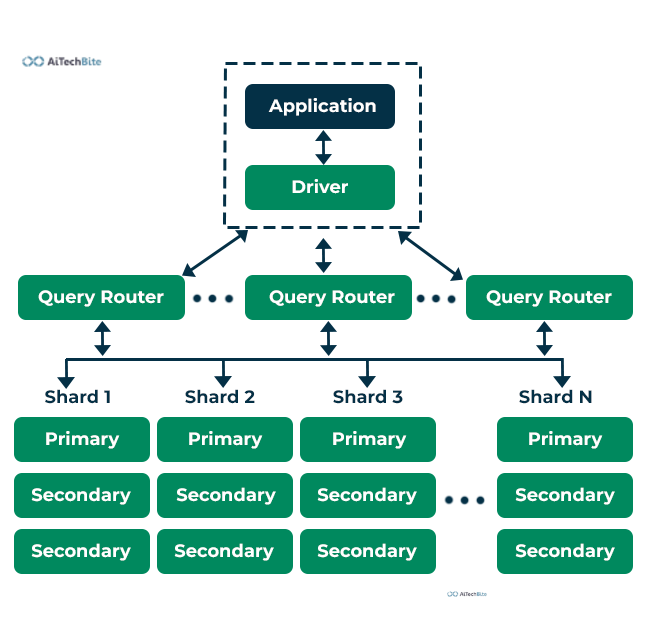
You can also add filters to the find()
command to retrieve specific documents. For instance, to find all students with the name “Vinod”, you would use:
db.students.find({name: "Vinod"})
These are just the basics of working with MongoDB databases and collections. As you progress, you’ll learn more advanced techniques, such as indexing, aggregation, and working with the MongoDB drivers in your application code.
Step 9 Exploring Further Resources ๐
To continue your MongoDB learning journey, be sure to check out the following resources:
- Thapa Technical MongoDB Complete Course in Hindi with Free Certification – AiTechBite
- GitHub – mongodb/mongo: The MongoDB Database
- Download MongoDB Community Server | MongoDB
- MongoDB Shell Download | MongoDB
- Download MongoDB Command Line Database Tools | MongoDB
- Subscibed Our YouTube Channel- AiTechBite
- MindIQ Online – Discover cutting-edge insights in AI, ML, cybersecurity, and more at Mindiq.online. Stay updated on the latest in tech!
- Creating Database in MongoDB | ft. Thapa Technical MongoDB Course in Hindi with Free Certification – YouTube
- How to Install and Setup MongoDB Server | Thapa Technical MongoDB Course in Hindi | Aitechbite – YouTube
Remember, the key to mastering MongoDB is to practice, experiment, and explore the vast resources available. Happy coding!