How to Take Input from User In Python – User Input in Python
BlogIn Python programming, handling user input is a fundamental skill that every programmer must master. User input allows programs to become interactive, enabling them to respond to user actions dynamically. This article will delve into various methods to take user input in Python, along with practical examples and explanations. We will also cover how to handle different data types and the importance of comments in your code.
Understanding User Input in Python
User input in Python can be captured using the built-in input()
function. This function reads a line from input, converts it to a string, and returns it. By default, all inputs received through this function are of string type, which is crucial to remember when performing operations on the input data.
Let’s look at a basic example:
user_input = input("Please Enter Something: ")
print("You entered:", user_input)
In this example, when the program runs, it prompts the user to enter something. After the user inputs their response and presses enter, the program prints the received input.
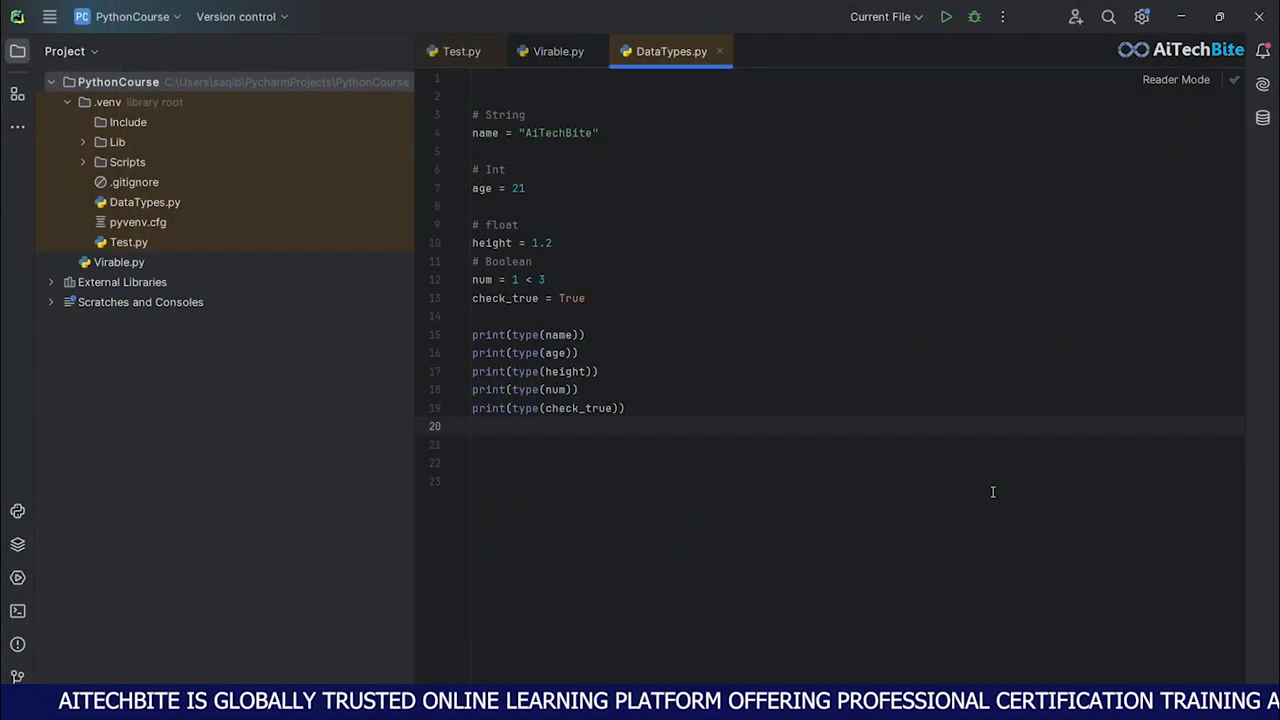
Taking Different Types of User Input in python
Although user input is received as a string, you may need to work with it as different data types, such as integers or floats. This requires type conversion, which can be done using Python’s built-in functions like int()
and float()
.
Example: Integer Input
Here’s how you can take an integer input from the user:
number = int(input("Enter a number: "))
print("You entered the number:", number)
In this example, the input is explicitly converted to an integer using the int()
function. If a user enters a valid integer, the program will display it. However, if the input is invalid (for instance, a letter), it will raise a ValueError.
Example: Float Input
Similarly, you can take a float input:
float_number = float(input("Enter a decimal number: "))
print("You entered the decimal number:", float_number)
In this case, the input will be converted to a float, allowing for decimal values. Again, invalid inputs will result in an error.
Handling Errors in User Input in python
To make your program robust, it’s essential to handle potential errors that may arise from user input. You can use a try-except
block to catch exceptions and provide feedback to the user.
Example: Error Handling
try:
number = int(input("Enter a number: "))
print("You entered the number:", number)
except ValueError:
print("That's not a valid number! Please enter an integer.")
In this example, if the user inputs a non-integer value, the program will catch the ValueError and prompt the user with a helpful message instead of crashing.
Using Comments in Python
Comments are an essential aspect of writing clean code. They help document your code and explain the purpose of various sections. In Python, you can add comments using the #
symbol for single-line comments or triple quotes for multi-line comments.
Single-Line Comments
A single-line comment can be added like this:
# This is a single-line comment
print("Hello, World!") # This prints a greeting
Multi-Line Comments
For multi-line comments, you can use triple quotes:
"""
This is a multi-line comment.
It can span multiple lines.
"""
print("Hello, World!")
Comments improve the readability of your code, making it easier for others (and yourself) to understand your logic later.
Combining Input, Output, and Comments
Let’s combine our knowledge of user input, output, and comments into a single Python program. In this example, we will create a simple calculator that takes two numbers as input and performs addition.
# Simple Calculator
# This program adds two numbers provided by the user
# Taking first number as input
num1 = float(input("Enter the first number: ")) # Input for first number
# Taking second number as input
num2 = float(input("Enter the second number: ")) # Input for second number
# Performing addition
result = num1 + num2
# Displaying the result
print("The sum of", num1, "and", num2, "is:", result)
This program prompts the user for two numbers, adds them, and prints the result. Comments are included to describe each step, enhancing code clarity.
Advanced User Input Techniques
Beyond basic input handling, Python offers advanced techniques for managing user input. You can use libraries such as argparse
for command-line arguments and tkinter
for graphical user interfaces.
Using Argparse
The argparse
library allows you to handle command-line arguments easily. Here’s a brief example:
import argparse
# Create a parser object
parser = argparse.ArgumentParser(description='A simple command-line calculator.')
# Add arguments
parser.add_argument('num1', type=float, help='First number')
parser.add_argument('num2', type=float, help='Second number')
# Parse arguments
args = parser.parse_args()
# Perform addition
result = args.num1 + args.num2
print("The sum is:", result)
This program will take two numbers as command-line arguments and print their sum.
Using Tkinter for GUI Input
If you want to create a graphical interface for user input, you can use the tkinter
library. Here’s a simple example:
import tkinter as tk
def calculate_sum():
num1 = float(entry1.get())
num2 = float(entry2.get())
result = num1 + num2
label_result.config(text="The sum is: " + str(result))
# Create the main window
window = tk.Tk()
window.title("Simple Calculator")
# Create input fields
entry1 = tk.Entry(window)
entry2 = tk.Entry(window)
# Create a button to calculate
button = tk.Button(window, text="Calculate", command=calculate_sum)
# Create a label for result display
label_result = tk.Label(window, text="")
# Layout
entry1.pack()
entry2.pack()
button.pack()
label_result.pack()
# Run the application
window.mainloop()
This code creates a simple GUI application where users can input two numbers, click a button, and see the result displayed.
Conclusion
Taking user input in Python is a fundamental skill that enhances the interactivity of your programs. By mastering the input()
function, handling different Data Types, managing errors, and writing meaningful comments, you can create robust and user-friendly applications. Whether you are building command-line tools or graphical applications and Web Applications, Python provides the necessary tools to handle user input effectively.
As you continue to learn Python, don’t forget to practice taking user input and handling it appropriately. For more advanced learning, consider exploring additional resources and courses available online, such as the Ultimate Python Course For Beginners In Hindi – AiTechBite.
Happy coding! 😊